Building Agentic Workflows with Mastra: A Tale of Autonomy and Self-Writing Interfaces
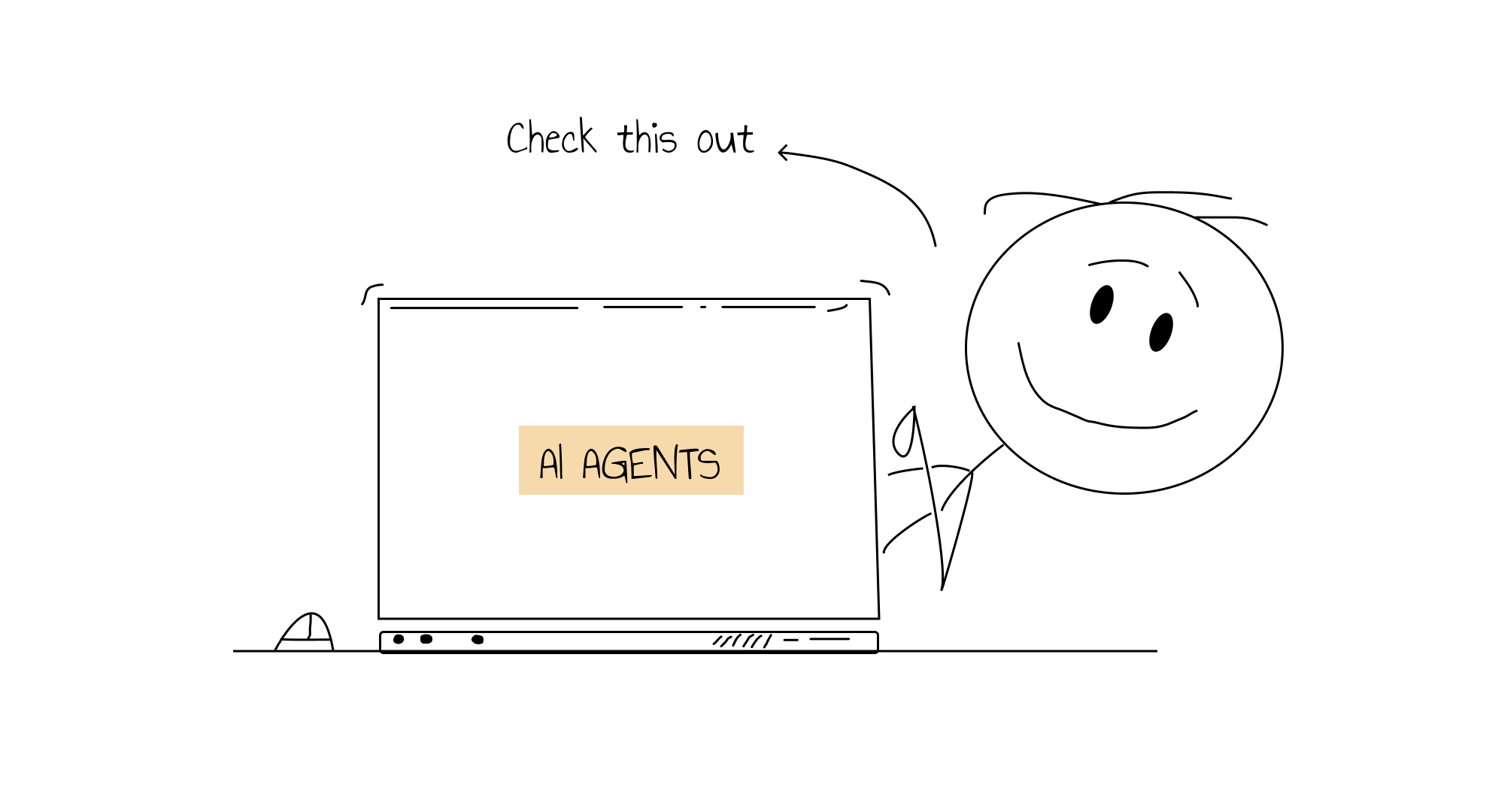
The lights in our office were almost all out, except for the one above my desk. It was 2 AM, and I was staring at my screen, watching something that felt like magic unfold before my eyes. Our UI was literally building itself.
When Our Interface Started Building Itself
It all started three weeks ago. We had been working on Vade Studio, our no-code platform that automates development workflows, when we hit a wall. The traditional approach to no-code builders required too much human intervention. We needed something more autonomous, something that could understand intent and translate it directly into interfaces.
That's when we discovered Mastra, an open-source TypeScript agent framework. What happened next changed everything about how we build software.
import { Agent } from "@mastra/core/agent";
import { openai } from "@ai-sdk/openai";
export const plannerAgent = new Agent({
name: "Project Manager",
instructions: "You analyze user requests and create execution plans for building interfaces in Vade Studio.",
model: openai("gpt-4o"),
});
Our first experiment was simple: could we create an agentic workflow that would build a landing page without human oversight? The results left us in disbelief.
The First Attempt Almost Crashed Everything
I still remember our first test. We had set up our planner agent to take a user's request and break it down into executable chunks.
// Our initial message handler
const response = await plannerAgent.generate([
{
role: "user",
content: "Create a landing page for a fitness app with a hero section, features list, and pricing table"
},
]);
What we got back was... interesting. Our planner created a beautiful, detailed plan - with components our system couldn't possibly build. We hadn't properly grounded the agent in our component library's capabilities.
// The fixed approach with proper constraints
const response = await plannerAgent.generate([
{
role: "system",
content: "IMPORTANT: Only use components from the Vade component library: Hero, CardList, PricingTable, ContactForm, Navbar, Footer."
},
{
role: "user",
content: "Create a landing page for a fitness app with a hero section, features list, and pricing table"
},
]);
The Moment Everything Clicked
The breakthrough came when we realized we needed a multi-agent system with specialized responsibilities:
// Our agent architecture
import { Mastra } from "@mastra/core";
import { z } from "zod";
// Project Manager: Creates execution plans
export const projectManager = new Agent({
name: "Project Manager",
instructions: "Analyze requirements and create execution plans with clear steps.",
model: openai("gpt-4o"),
});
// Designer: Handles UI/UX
export const designer = new Agent({
name: "Designer",
instructions: "Create compelling UI/UX designs using only Vade components.",
model: openai("gpt-4o"),
});
// Data Architect: Handles data and workflows
export const dataArchitect = new Agent({
name: "Data Architect",
instructions: "Create data models, integrate APIs, and design workflows.",
model: openai("gpt-4o"),
});
// DevOps Lead: Deployment and monitoring
export const devOpsLead = new Agent({
name: "DevOps Lead",
instructions: "Handle deployment and monitoring operations.",
model: openai("gpt-4o"),
});
// Register all agents
export const mastra = new Mastra({
agents: { projectManager, designer, dataArchitect, devOpsLead },
});
We defined clear roles, and more importantly, we created structured outputs for each agent using Zod schemas:
// Schema for UI component operations
const uiOperationSchema = z.object({
componentType: z.enum(["Hero", "CardList", "PricingTable", "ContactForm", "Navbar", "Footer"]),
properties: z.record(z.any()),
children: z.array(z.any()).optional(),
position: z.object({
x: z.number(),
y: z.number(),
}),
});
// Designer agent with structured output
const designResponse = await designer.generate(
[
{
role: "user",
content: `Create a hero section based on this plan: ${planDetails.sections.hero}`
}
],
{
output: uiOperationSchema,
}
);
console.log("Component to create:", designResponse.object);
The Workflow That Built Itself
The magic of our system came alive when we connected everything into a workflow:
// A simplified version of our agentic workflow
async function buildInterfaceFromIntent(userIntent) {
// Step 1: Project Manager creates a plan
const planResponse = await projectManager.generate([
{ role: "user", content: `Create a plan for: ${userIntent}` }
], {
output: planSchema
});
const plan = planResponse.object;
// Step 2: Designer creates UI components
const uiComponents = [];
for (const section of plan.sections) {
const designResponse = await designer.generate([
{ role: "user", content: `Design the ${section.name} section with these requirements: ${section.requirements}` }
], {
output: uiOperationSchema
});
uiComponents.push(designResponse.object);
}
// Step 3: Data Architect creates data models and workflows
const dataModelResponse = await dataArchitect.generate([
{ role: "user", content: `Create data models and workflows for: ${userIntent}` }
], {
output: dataModelSchema
});
// Step 4: Apply operations to the UI
applyUIOperations(uiComponents);
return {
uiComponents,
dataModel: dataModelResponse.object
};
}
The Unexpected Side Effects
Not everything went smoothly. There was that time when our designer agent decided that every single component needed to be "eye-catching" and "pop":
// What we got back once
{
componentType: "Hero",
properties: {
backgroundColor: "#FF00FF", // Bright magenta
fontFamily: "Comic Sans MS",
fontSize: "72px",
animation: "bounce 2s infinite"
},
position: { x: 0, y: 0 }
}
We quickly added some design constraints after that.
What We Learned About Agentic Workflows
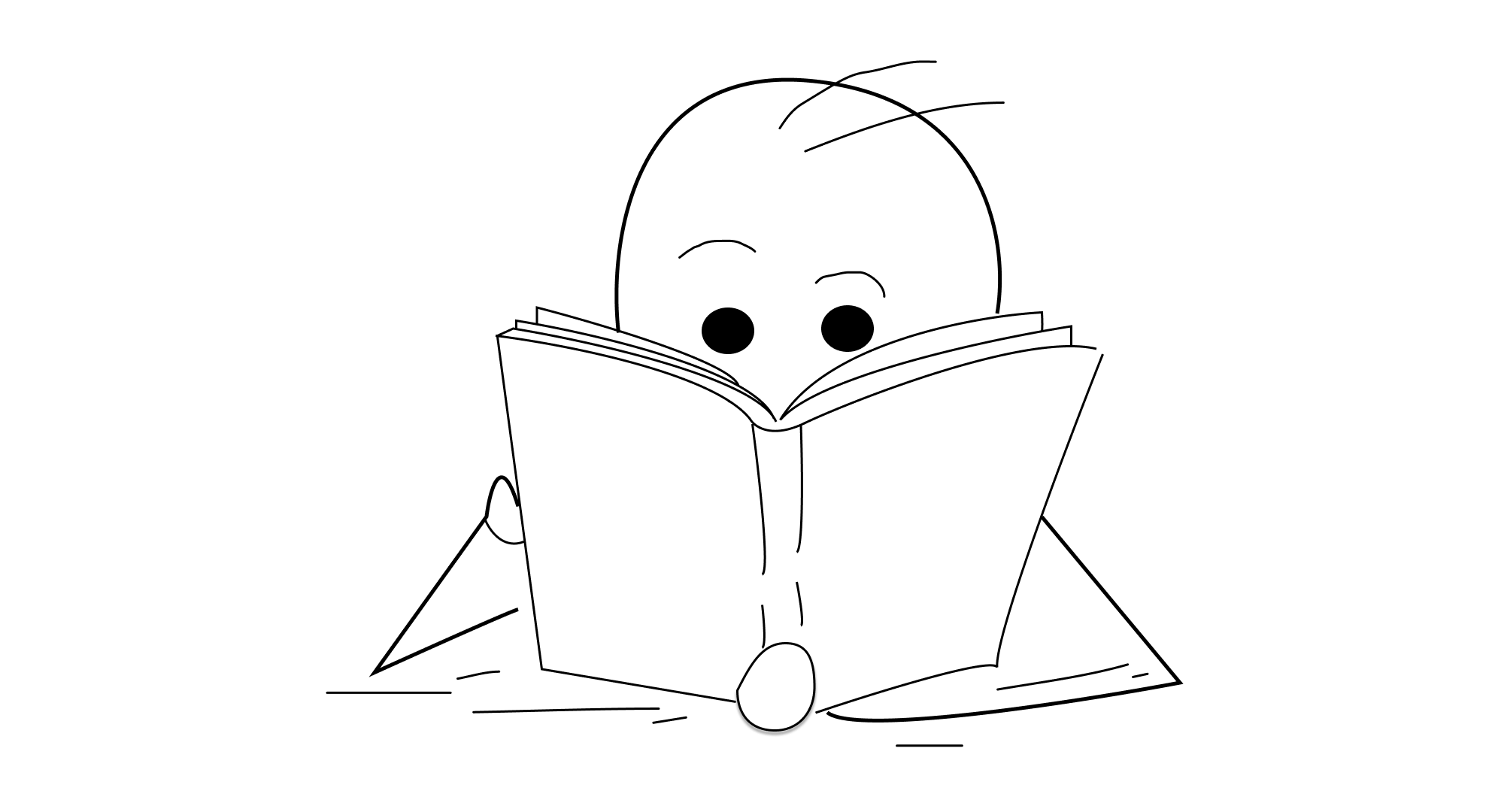
Building with Mastra taught us some fundamental truths:
- Agents need clear boundaries and well-defined capabilities
- Structured outputs are non-negotiable for reliable operations
- Each agent should have a singular focus and clear instructions
- The communication between agents is as important as the agents themselves
The Unconventional Architecture That Worked
Our most controversial decision was to have our agents generate operations rather than direct code or configurations. Many advised us to have the agents generate React components or configuration JSON directly.
Instead, we built an operation translator that took high-level directives like:
{
"id": "string", // Unique operation identifier
"type": "string", // Operation type
"group": "string", // Grouped actionables, It could be same for different operations for each section. It should be two to three words
"message": "string", // Short quirky and interesting description about what is happening
"context": {
"engine": "string", // Engine identifier
"workspace": "string" // Workspace identifier
},
"data": {
"source": [], // Array of source components
"target": {} // Target component definition
}
}
And translated them into actual UI changes. This seemingly indirect approach gave us tremendous flexibility and reliability.
The Surprising Power of Chain-of-Thought
One unexpected learning was how much the quality improved when we implemented chain-of-thought reasoning. By having our agents explicitly reason through their decisions, we got dramatically better results:
const plannerPrompt = `
First, analyze what type of application this would be.
Second, identify the key sections needed for this type of application.
Third, determine the data requirements for each section.
Finally, create a step-by-step plan for building this application.
User request: ${userRequest}
`;
const response = await projectManager.generate([
{ role: "user", content: plannerPrompt }
]);
When It All Came Together
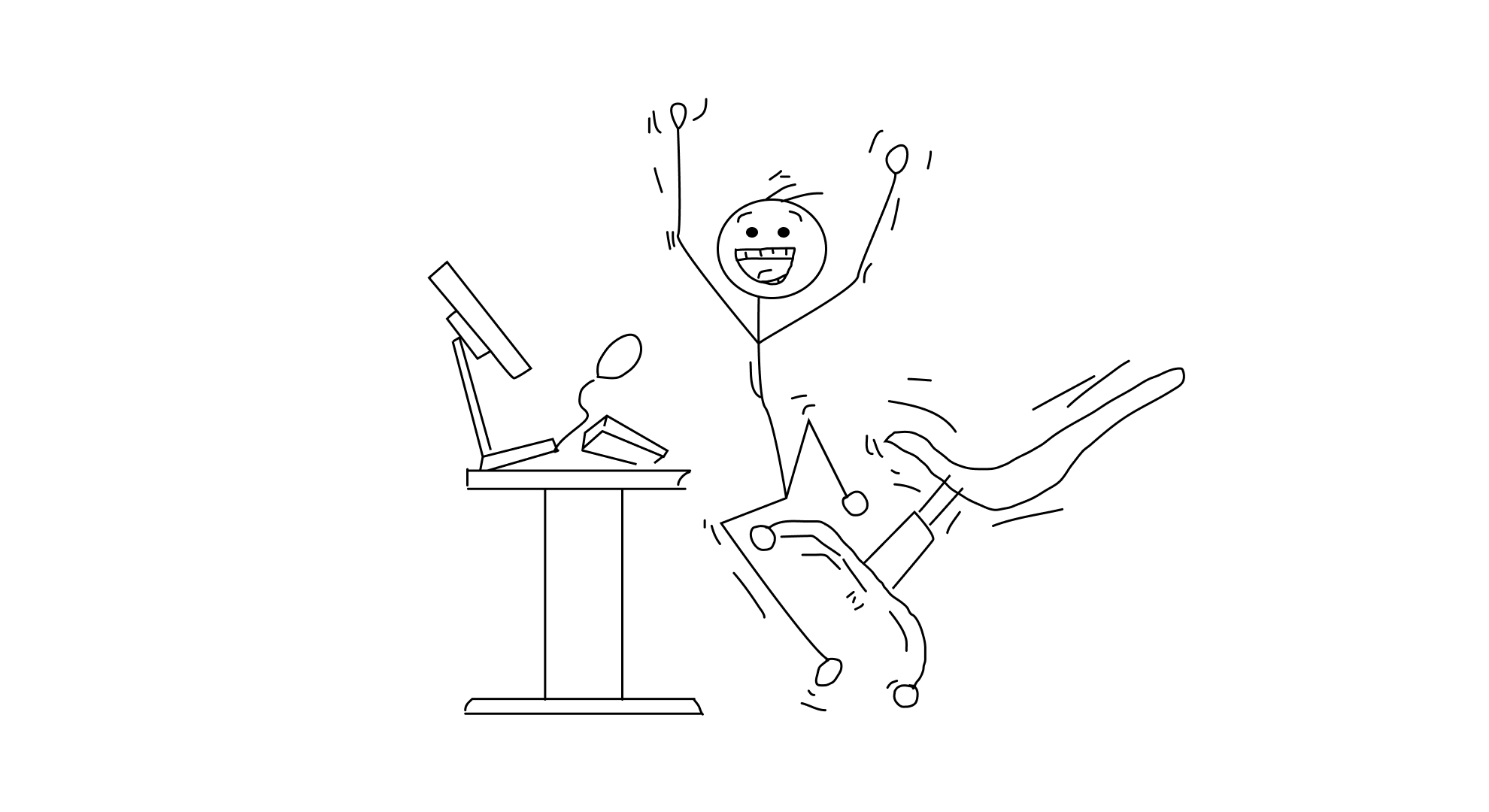
There was a moment, after weeks of work, when we demoed the system to our team. We typed in a simple request: "Create a customer feedback collection page with a form and testimonial display."
Within seconds, our agents sprang into action. The project manager created a plan, the designer crafted the UI components, the data architect established the database schema, and the DevOps lead prepared deployment instructions.
Two minutes later, a complete feedback collection page appeared on screen—no human intervention required.
The room went silent, then erupted in applause. This wasn't just a demo; it was a glimpse into the future of software development.
The Road Ahead
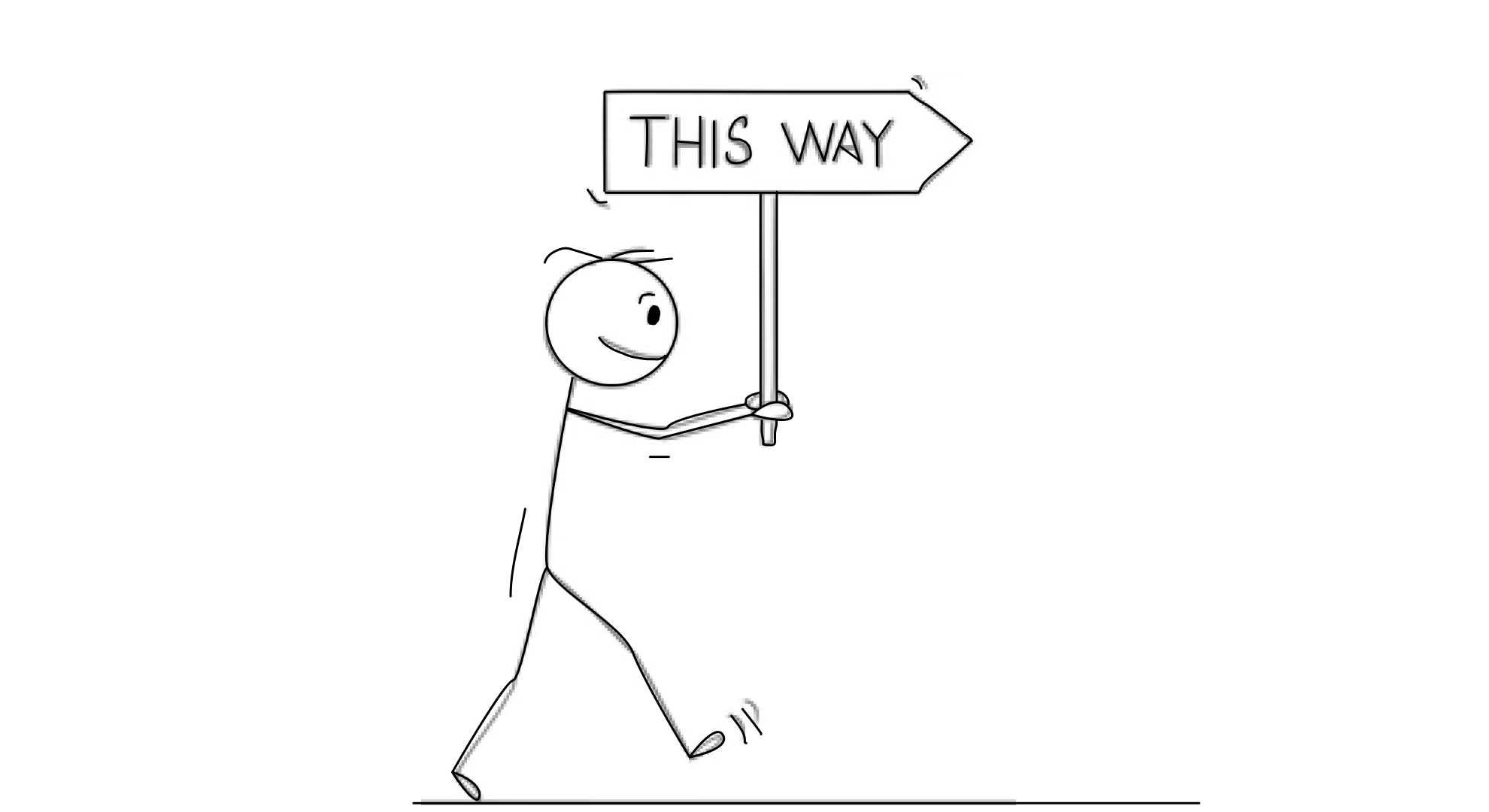
We're still early in our journey with Mastra and agentic workflows. There's much to improve—reliability, edge cases, and giving agents more context awareness.
But one thing is clear: the traditional way of building software is changing. With frameworks like Mastra and platforms like Vade Studio, we're moving toward a world where developers describe what they want to build, and AI agents handle the implementation details.
It's not about replacing developers—it's about elevating what they can create. As we continue refining our agentic workflows, we're excited to see where this journey takes us, and how it transforms the world of software development.
The future isn't just code that writes itself—it's interfaces that build themselves based on human intent. And we're just getting started.